(New!) - GitHub Page of the Core plugin: https://github.com/kingplugins/marketking-core
In this article, we'll try to list everything you need to know when developing with MarketKing:
- Edit or overwrite dashboard or page templates
- Available plugin functions
- Available plugin hooks
- How to integrate a plugin (Example)
- How to add a custom link or page to the MarketKing dashboard
How to edit or overwrite plugin page templates
MarketKing's dashboard pages, as well as the stores page and individual store pages, can be edited by copying the PHP templates to your theme or child theme. For more, see our dedicated guide on how to edit pages & templates.
Available plugin functions
The plugin has a variety of global functions that you can use anywhere on your site. These can all be found in marketking-core/includes/class-marketking-core-helper.php
Example:
$vendor_rating = marketking()->get_vendor_rating($vendor_id);
The above function will return the aggregate review score based on the vendor ID
echo marketking()->get_vendor_rating(45); - this will display the rating of the vendor with the USER ID of 45
Available functions:
- marketking()->get_store_name_display($vendor_id) - returns the Store Name, ready to be displayed
- marketking()->get_store_link($vendor_id) - returns the store url based on the vendor id
- marketking()->get_product_vendor($product_id) - returns the vendor id based on the product id
- marketking()->get_order_vendor($order_id)
- marketking()->is_vendor($user_id) - returns true or false, based on whether the user is a vendor
- marketking()->get_vendor_email($vendor_id)
- marketking()->get_vendor_rating($vendor_id)
- marketking()->get_vendor_products($vendor_id, $count = false) - if count is set to false, it returns an array of product ids of that vendor; if set to true, it returns the number (count) of products that vendor has
- marketking()->get_vendors_in_cart() - returns array of vendor ids whose products are currently in cart
- marketking()->get_vendors_of_order($order_id) - returns array of vendor ids for an order
- marketking()->get_number_of_followers($vendor_id) - returns how many followers a vendor has
- marketking()->get_vendor_order_number($vendor_id) - returns how many orders that vendor has
- marketking()->store_url_exists($store_url) - returns true or false, based on whether the store url (store slug) exists.
- marketking()->is_vendor_store_page() - returns true or false, based on whether the current page is a vendor store page
- marketking()->get_store_content_by_url($store_url) - returns the store page content based on the store url (slug)
- marketking()->get_vendor_id_by_url($store_url)
- marketking()->vendor_can_multiple_categories($vendor_id) - returns true or false, based on whether the vendor has permission to select multiple categories per product
- marketking()->vendor_can_linked_products($vendor_id)
- marketking()->vendor_can_new_attributes($vendor_id)
- marketking()->vendor_can_product_category($vendor_id, $category_id) - returns true or false based on whether, a vendor can post in that specific category
- marketking()->vendor_can_publish_products($vendor_id) - returns true or false, based on whether the vendor can publish products directly
These are just some of the functions available, and this is not a complete list. There are many more functions in the file mentioned above, and more will continue to be added. We recommend you check the helper file directly. If there's a function you need, feel free to contact our support - we may be able to add it if it doesn't already exist.
Available plugin hooks
Here are some of the plugin's hooks:
Action hooks:
- marketking_before_save_product($product_id, $vendor_id) - runs before a product is saved
- marketking_after_save_product($product_id, $vendor_id) - runs after a product is saved
- marketking_edit_product_after_tags($post) - runs in the vendor dashboard, after the tags element. Can be used to insert custom items in the vendor dashboard product page
Filters:
- marketking_show_actions_my_orders_page(true) - shows or hides actions on the my orders page in the dashboard
- marketking_dashboard_products_show_stock_qty(true) - shows product stock qty in the dashbaord
- marketking_store_name_max_length(25) - maximum allowed length for a vendor store name
- marketking_default_products_number(12) - number of products shown by default on a vendor store page
- marketking_vendor_reviews_per_page(5) - number of vendor reviews shown per page
There are many more actions and filters throughout the plugin. It is best to check files directly. Feel free to contact our support team and we'll be happy to assist with info if you're looking for anything in particular,
How to integrate a plugin
Generally speaking, most plugin integrations will have to do with extending a plugin so that its functionalities become available for vendors in the vendor dashboard. Otherwise, since MarketKing uses regular WooCommerce products and features, most plugins are already perfectly compatible.
To integrate a plugin with MarketKing in order to show its features in the vendor dashboard, there are 2 main steps:
- Display that plugin's features in the vendor dashboard
- Enqueue that plugin's styles and scripts in the vendor dashboard
Example: QR Code Plugin integration ( https://wordpress.org/plugins/qr-code-woocommerce/ )
This plugin adds a QR code on each product page backend, so it can be printed and displayed. We want to extend this so that vendors can also see this QR on their dashboards.
This is what is seen on the product page backend:
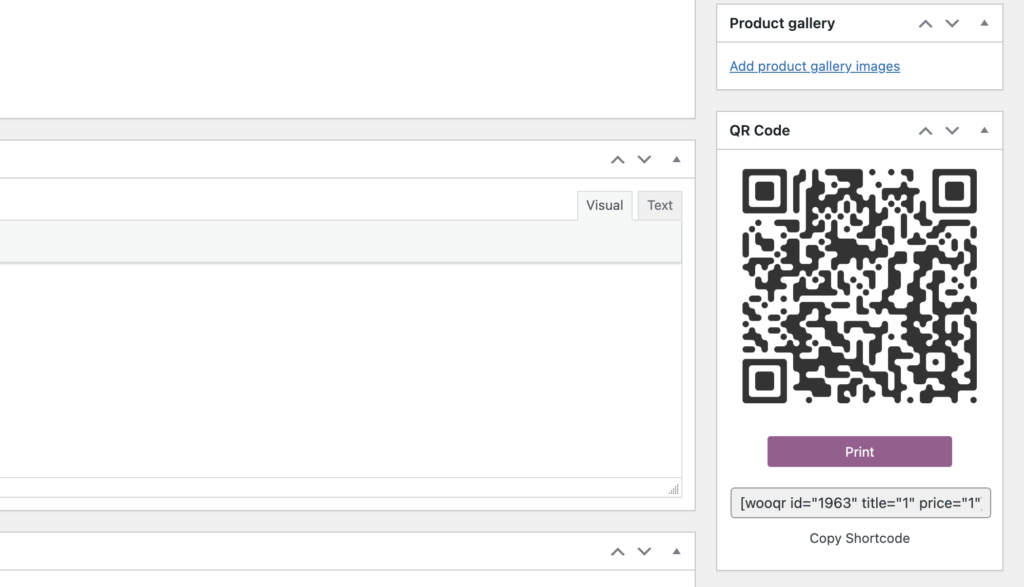
We need to make the above QR also available to vendors.
First of all, we want to display it on the product edit page in the vendor dashboard. The file for this is in marketking-core/public/dashboard/edit-product.php
By looking at this file we find the marketking_edit_product_after_tags($post) hook that we can use.
Then we add the following code to the site or plugin:
add_action('marketking_edit_product_after_tags', function($post){
$productid = $post->ID;
if(class_exists('WooCommerceQrCodes')){
?>
<div class="row">
<div class="col-xxl-3 col-md-6 marketking_card_gal_cat_tags">
<div class="code-block marketking_cattag_card"><h6 class="overline-title title"><?php esc_html_e('QR Code','marketking-multivendor-marketplace-for-woocommerce');?></h6>
<?php echo do_shortcode('[wooqr id="'.esc_attr($productid).'" title="1" price="1"]'); ?>
</div>
</div>
</div>
<?php
}
}, 10, 1);
Let's try to dissect the above code:
- First we get the product ID from the post object
- We check if that plugin is active by checking for its class via class_exists
- Then we add a row to display the QR code in, and we use that plugin's QR display shortcode, replacing the product ID with our variable
Now we have an element there in the vendor dashboard, but it does not display correctly as a QR code yet. In the vendor dashboard now we see:
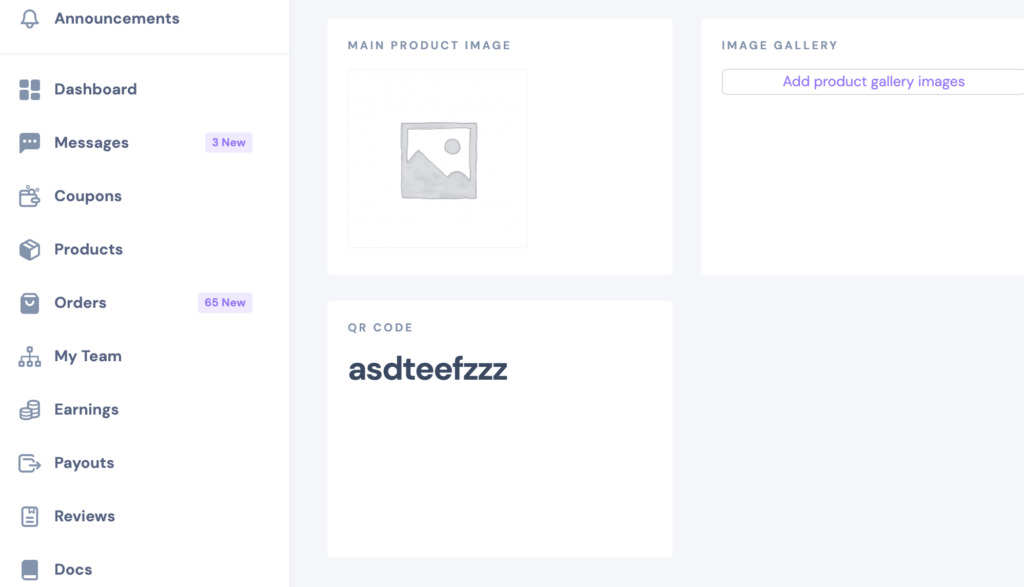
By opening the JavaScript console we see the following error:
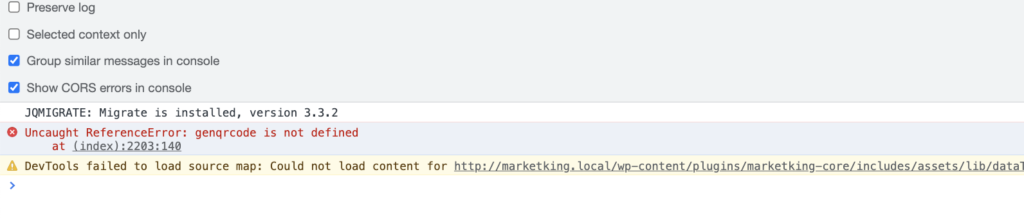
The problem is that the genqrcode script does not exist. We open the QR code plugin files and we search across all files (CTRL + SHIFT + F in some programs) for 'genqrcode'. This helps us find where the scripts and styles of that plugin are enqueued on the frontend.
All that's now left to do is add those to MarketKing:
To do that we use:
add_action('marketking_dashboard_head', function(){
add_action('wp_print_styles', function(){
// add styles here
});
add_action('wp_print_scripts', function(){
// add scripts here
});
});
Finally, we copy paste from the the QR files and we get:
add_action('marketking_dashboard_head', function(){
if (class_exists('WooCommerceQrCodes')){
add_action('wp_print_styles', function(){
global $WooCommerceQrCodes;
$wooqr_options = array(
'qr_options' => get_option('wooqr_option_name')
);
wp_enqueue_style('wcqrc-product', $WooCommerceQrCodes->plugin_url . 'assets/css/wooqr-code.css', array(), $WooCommerceQrCodes->version);
wp_enqueue_style('qrcode-style', $WooCommerceQrCodes->plugin_url . 'assets/admin/css/style.css', array('jquery'),
$WooCommerceQrCodes->version);
});
add_action('wp_print_scripts', function(){
global $WooCommerceQrCodes;
$wooqr_options = array(
'qr_options' => get_option('wooqr_option_name')
);
wp_enqueue_script('qrcode-qrcode', $WooCommerceQrCodes->plugin_url . 'assets/common/js/kjua.js', array('jquery'),
$WooCommerceQrCodes->version);
wp_enqueue_script('qrcode-createqr', $WooCommerceQrCodes->plugin_url . 'assets/common/js/createqr.js', array('jquery'),$WooCommerceQrCodes->version);
wp_localize_script( 'qrcode-createqr', 'wooqr_options', $wooqr_options );
});
}
});
Now the styles and scripts have been enqueued and we can see it on the dashboard;
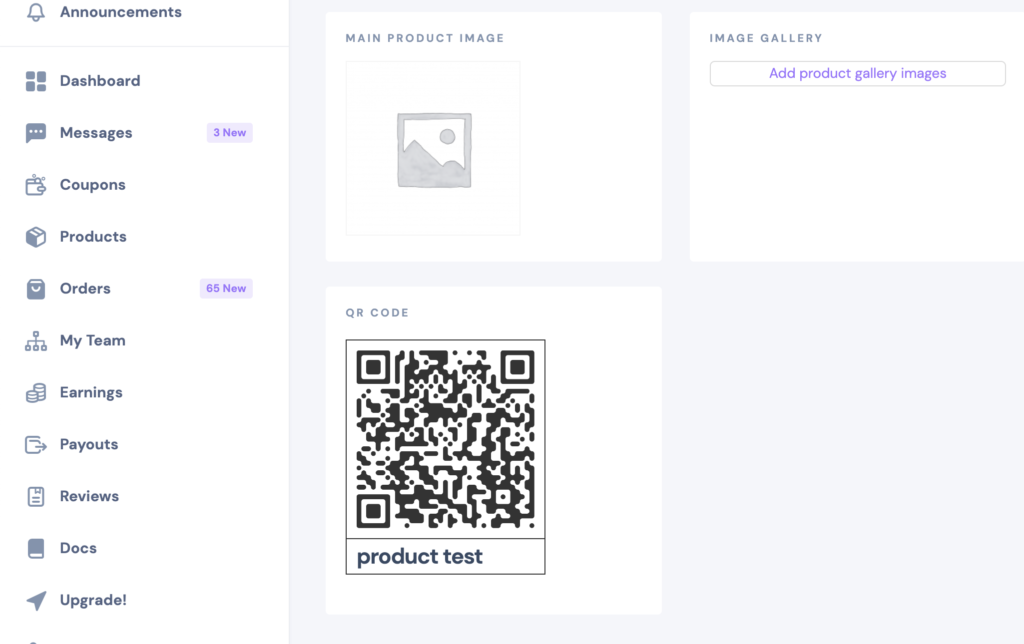
How to add a custom link or page to the MarketKing dashboard
To add a custom link, use this code snippet:
add_action('marketking_extend_menu', function($menu){
?>
<li class="nk-menu-item">
<a href="<?php echo 'https://test.com';?>" class="nk-menu-link">
<span class="nk-menu-icon"><em class="icon ni ni-book-fill"></em></span>
<span class="nk-menu-text">New Test Item</span>
</a>
</li><!-- .nk-menu-item -->
<?php
}, 10, 1);
Result will be:
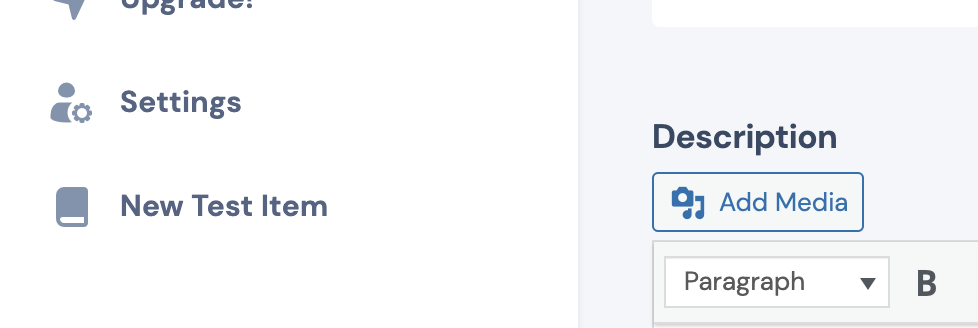
To change the Icon of that, replace book-fill with one of the options from here: https://dashlite.net/demo2/components/misc/nioicon.html
The above will represent a simple link.
To add a page with content rather than a simple link, use this snippet:
add_action('marketking_extend_menu', function($menu){
?>
<li class="nk-menu-item">
<a href="<?php echo esc_attr(get_page_link(apply_filters( 'wpml_object_id', get_option( 'marketking_vendordash_page_setting', 'disabled' ), 'post' , true))).'newpage';?>" class="nk-menu-link">
<span class="nk-menu-icon"><em class="icon ni ni-navigate-fill"></em></span>
<span class="nk-menu-text"><?php echo 'New Page!';?></span>
</a>
</li><!-- .nk-menu-item -->
<?php
}, 10, 1);
add_action('marketking_extend_page', function($page){
if ($page === 'newpage'){
?>
<div class="nk-content marketking_products_page">
<div class="container-fluid">
<div class="nk-content-inner">
<div class="nk-content-body">
<div class="nk-block-head nk-block-head-sm">
<div class="nk-block-between">
<div class="nk-block-head-content">
<h3 class="nk-block-title page-title"><?php esc_html_e('New Page!','marketking-multivendor-marketplace-for-woocommerce'); ?></h3>
</div><!-- .nk-block-head-content -->
</div>
</div>
<div>
New content here!
</div>
</div>
</div>
</div>
</div>
<?php
}
});
You can then see your new page:
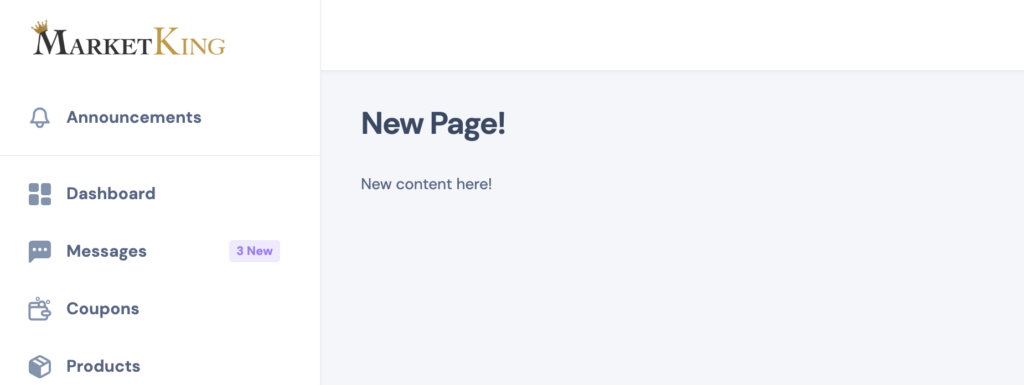